In mobile application development, accessing data from an external source is fundamental to development process. Keeping data separate from the client makes the applications run faster.
In this article, I’ll let you know the efficient way of fetching data from an API.
What is an API?
Application Programming Interface (API) acts like a bridge between different software applications to communicate with each other. It’s a set of rules that help the interaction between different applications.
Why APIs are important?
Since one application has to go through the API to communicate with the other, the API plays its role in the following context:
- We ensure that only authorized apps (clients) access the data on the server. It is ensured that the information is safe and in good hands.
- We separate the data and mobile (and other) applications from each other for their enjoyable working.
- APIs make high data & volume requests easier.
- We make programs efficient by using APIs.
What is an HTTP Request?
When a mobile application (client) tries to send a message to the server, this message is called an HTTP (Hypertext Transfer Protocol) request. Server responses to the request with status information and data requested.
We have many HTTP request methods that we use to make applications interact. But here I’m only briefing on the most used ones:
- GET request: this method retrieves information from an endpoint in the API. Getting the user’s information from a database is an example of GET request.
- POST request: this method writes new information to a specific endpoint. It’s just like submitting a form or creating a table of information in the database.
- PUT request: using this method, we can update existing information. You can think about changing the username of a user in his profile.
- DELETE request: this request removes the existing data from an endpoint, e.g, deleting a user account from a database.
Fetching Data from an API in React Native
I have a React component ‘ApiCalls‘ in which useState hook is declared to store the data.
In the getMovies function, the JavaScript’s fetch() method is used to retrieve the movies information from the given URL. The fetch() method accepts a URL from where the data is being retrieved. On successful fetching, this data is pushed to the state varible.
The useEffect() hook calls our function automatically when the component mounts.
import React, { useEffect, useState } from "react"; import { ActivityIndicator, FlatList, Text, View } from "react-native"; const ApiCalls = () => { const [isLoading, setLoading] = useState(true); const [data, setData] = useState([]); const getMovies = async () => { try { const response = await fetch("https://reactnative.dev/movies.json"); const json = await response.json(); setData(json.movies); } catch (error) { console.error(error); } finally { setLoading(false); } }; useEffect(() => { getMovies(); }, []); return ( <View style={{ flex: 1, padding: 24 }}> {isLoading ? ( <ActivityIndicator /> ) : ( <FlatList data={data} keyExtractor={({ id }) => id} renderItem={({ item }) => ( <Text> {item.title} - {item.releaseYear} </Text> )} /> )} </View> ); }; export default ApiCalls;
You’ll see the data shown using FlatList as:
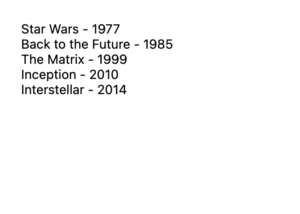
This article allows you to fetch movies list with their released years using the fetch(). Hopefully, you have enjoyed this article. Share it with other developers.
Follow our social channels for such valuable content.
Thank you for reading💖